Five Lessons from refactoring StackOverflow code that will make your JS coding journey 253% easier
A talk given at BrisJS on the top five things that will make the biggest difference to your JS programming, including a live refactoring of a StackOverflow question.
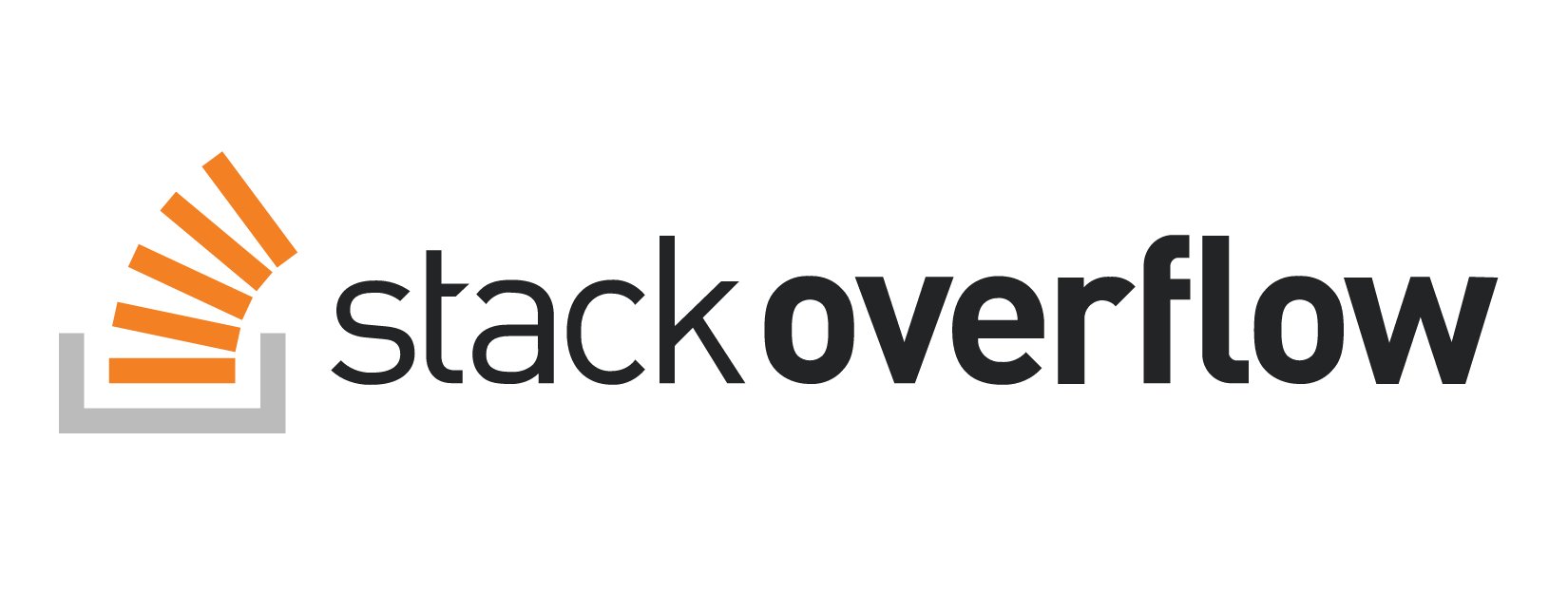
The GitHub repo with the code for this talk is here.
You can watch the video of the talk, including the live refactoring, here on YouTube, or below.
Background
In early 2020, I noticed that my worldwide ranking on StackOverflow had dropped, and I was only in the top 25% in the world.
Unacceptable.
I went on a burn, and I’m now back in the top 0.60% - where I belong. ;-)
When you expose yourself to a vast amount of data in a short period of time, patterns start to emerge.
I noticed that 80% of questions in the JavaScript tag on StackOverflow had the same fundamental problems.
They required much deeper refactors than the answer “How do I get this code to work”, so they were really out of scope for an answer.
To capture them somewhere, I started a category on my blog called “StackOverflowed” where I described the deeper issues, and refactored them.
In the BrisJS talk, I cover the top five things that, I believe, will most improve your JS programming; and did a live refactor of a question from StackOverflow.
The Five Things (and one bonus)
0. Let vs Var vs Const
Use const
for everything. Anything that is left is part of a state machine, and should be encapsulated.
Understand that arrays and objects are object references, and should be declared as const
. It’s a common misconception that the array contents are immutable if declared as const
.
The object reference of an array is unchanged, even if you modify the contents of the array. And if you push to an array, it’s a state machine - so you should refer to the next point.
See the article Shun the Mutant on my blog.
1. Global Mutable State
Any genuine variables in a program represent actual mutable state - which is rare in a program. Things like time, the state of the network connection, a current score, are variables. And they belong in state machines with immutable-value-returning interfaces. See 01-global-mutable-state.js, and the article Avoid Global State on my blog.
2. Loops
Loops are state machines. When you solve a problem using a loop, now you have two problems.
Mostly, people thing “I have to do something to every element in this array”, and construct a loop.
However, what you need to do is “do something to each element in this array” - which means a function and Array.map.
See the article Why Array.map on my blog.
3. Data transformation
Niklaus Wirth wrote the classic book “Algorithms + Data Structures = Programs” in 1976.
A lot of programming is transforming data structures. If you are doing a lot of complex transformations, revisit the data structures.
If you don’t control input data structures (for example, you consume them for a web service you don’t control), then you will have to transform them.
If you find yourself writing a loop and using Array.push, you should be doing Array.map.
Correctly and accurately naming your data structures, intermediate data structures, and transformer functions will make the Dao clear.
See the article Providing a Semantic API on my blog.
4. Promises
A lot of JS programming is async. A common problem is “retrieve some data from a service, make some determinations based on that data, then retrieve some other data, return that data to the caller”.
In this case, you want Promise.all, Array.map, and Array.filter.
See the article Handling Failure and Success in an Array of Asynchronous Tasks on my blog.
Bonus: Naming
80% of programming is naming things. When things are correctly named, the Dao becomes clear.
Live Refactoring a StackOverflow Question
In the BrisJS talk, I refactored a StackOverflow question live. It was the third question on the page in the JavaScript tag when I went on there, and demonstrates many of the factors that I cover.
The question that I refactored is here.
The code for the refactor is here.
You can see the question in demo.js, along with stub code to mock the services returning the remote data structures.
To run it: node demo.js
.
A refactor with no loops and no variables is in refactor.js.
To run it: node refactor.js
.